Ok so this is to be a semi brief overview of the networking protocol and related systems as we currently under stand them.
This is mirrored on the wiki at: https://github.com/themeldingwars/Documentation/wiki and is here for easier discussion and feedback.
Login and Zone Selection
This will just be a brief overview of this part, there is more to this that can be covered later.
The initial steps are done over HTTPS to a web server.
- User sends a log in request with user name / password
- If authenticated the web server sends back a character list, this gets you to the choose a character screen.
- When the user clicks the "Enter World" button another HTTPS request is made to get an "Oracle Ticket"
- The server sends back an oracle ticket like below.
"matrix_url": 127.0.0.1,
"ticket": "A base64 encoded data string, contents not known as of yet",
"datacenter": "localhost",
"operator_override": {
"ingame_host": "https://indev.themeldingwars.com/ingame_host",
"clientapi_host": "https://indev.themeldingwars.com/clientapi"
},
"session_id": "8360c86c-a3d0-11e4-9e16-c074c1266b6d",
"hostname": "localhost",
"country": "GB"
The key bit here is the matrix_url
this is the ip that the game client will now start sending packets to over UDP on port 25000
Note: We have a simple test web API up for local server testing at https://indev.themeldingwars.com this server just returns an Oracle ticket pointed to 127.0.0.1:25000
To use open your Firefall.ini in the games install and add
[Config]
OperatorHost= "indev.themeldingwars.com"
Game Server Connection
The data is in Big Endian
Handshake
Now that the client knows where to connect it has to establish a connection with that game server.
This is all done over UDP and the game uses a custom networking protocol over UDP for reliability when it is deemed necessary.
The command format for these handshake messages is:
[uint] [char[4]]
---------- -----------
SocketID Id for msg
00 00 00 00 50 4F 4B 45
For the matrix handshake the socket id is always 0
The first packet that the client sends a POKE
message to the server to start off the connection.
(You can use matrix_connectiontest ip
in the console to test the handshaking and a simple connection)
The flow is
[Client] [Server]
POKE ->
<- HEHE
KISS ->
<- HUGG
(Yes they really are called HEHE, KISS, POKE and I love it :D) (and aperantly a hug comes after the kiss)
Below are the packets send and received as part of the handshake.
POKE (Client)
[uint] [char[4]] [uint]
---------- ----------- ----------------
SocketID Id for msg Protocol Version
00 00 00 00 50 4F 4B 45 00 04 B9 68
HEHE (Server)
[uint] [char[4]] [uint]
---------- ----------- ----------------
SocketID Id for msg SocketID
00 00 00 00 50 4F 4B 45 F1 07 12 8E
KISS (Client)
[uint] [char[4]] [uint] [ushort]
---------- ----------- ---------------- ----------------
SocketID Id for msg SocketID Streaming Protcol
00 00 00 00 50 4F 4B 45 F1 07 12 8E 4C 5F
HUGG (Server)
[uint] [char[4]] [ushort] [ushort]
---------- ----------- ---------------- ----------------
SocketID Id for msg SequenceStart GameServerPort
00 00 00 00 50 4F 4B 45 F7 A1 D2 65
ABRT
[uint] [char[4]]
---------- -----------
SocketID Id for msg
00 00 00 00 41 42 52 54
Used to end a connection
Main Connection
After a successful handshake the client will then start to communicate to the game server on the port that was assigned in the above handshake. eg the game will start sending packets to 127.0.0.1:53861
and the client will receive packet on port that the first matrix handshake message was received from.
All packets to and from the server start with a uint
for the socket id that was assigned from the handshake. (Again if the id is 0 is a matrix handshake message).
Below is the packet header:
[uint] [2bits] [2bits] [1bit] [11bits]
---------- --------- ------------ ------------ ------------
SocketID Channel Resend Count Is Splitted Length
TODO: Check
UDP packets have a max size and so if a message being sent is bigger than this size it will have to be split across multiple packets.
If a packet is splitted then the Is Splited
will be 1
, split packets are per channel and not global across them.
If a channel comes across a split packet then that stream is blocked until all the all the fragments for it are received and can be assembled back into the full packet.
If a packet is marked as splited the data for that should be held in a buffer and the next message with the sequence number directly after should be appended. This process should be repeated until the next packet in sequence that doesn't have the Is Splitted
set is encountered, this marks the end of that split packet.
All the buffered packet data up untill but not including this should be reassembled to form the full packet data.
There are 4 different channels that a message can get sent over Channel
indicates what one the message is using.
Channel 1 and 2 are reliable while channel 0 and 3 are unreliable.
We need to branch the logic for parsing a packet here to handle the channel types.
Channel 1, 2 and 3
All of these channels have another ushort
for the sequence number for that packet.
The sequence number is per channel and per direction. eg sending on channel 1 will have a different sequence number than sending on channel 2.
Channel 1 and 2
Since these are both reliable channels we need to check the Resend Count
, if this is greater than 0 than we need to xor the data to get the correct values. and ofc its not a static value to xor against, we use the Resend Count
to index a preset list of xor values.
Below is the list of xor values to use.
byte[] xors = new byte[] { 0x00, 0xFF, 0xCC, 0xAA };
eg if the Resend Count
is 1 then we would XOR all the data after the Sequence Number
with 0xFF (xors[Resend Count]
)
Channel 2 and 3
The next part of the header is common for both of these channels.
[byte] [byte[6]] [byte]
------------- --------- ------------
Controller ID Entiy ID Msg ID
Note: This is the same as the player CID that can be gotten from the LUA API only with the first and last byte overwritten.
The data after this header is packet specific.
We can route to the correct handler with the controller ID and the Msg ID
Note: At this point the data here is very similar to that contained in the replay files
Below is the way to route the packet handling:
click to show
Controller packet routing
prod-1962 packet list
ControllerID = ?
MessageId = ?
if(ControllerID < 240)
Switch(ControllerID-1)
{
case 0 -> 13
Switch(MessageId)
{
83 firefall::Character::MarketRequestComplete
84 firefall::Character::ReceiveWeaponTweaks
85 firefall::Character::TookDebugWeaponHitPublic
86 firefall::Character::TookDebugWeaponHit
87 firefall::Character::DebugWeaponStats
88 firefall::Character::RewardInfo
89 firefall::Character::ProgressionXpRefresh
90 firefall::Character::ReceivedDeferredXP
91 firefall::Character::PublicCombatLog
92 firefall::Character::PrivateCombatLog
93 firefall::Character::AnimationUpdated
94 firefall::Character::RaiaNPCDebugging
95 firefall::Character::WeaponProjectileFired
96 firefall::Character::AbilityProjectileFired
97 firefall::Character::ProjectileHitReported
98 firefall::Character::Stumble
99 firefall::Character::QuickChat
100 firefall::Character::ProximityTextChat
101 firefall::Character::JumpActioned
102 firefall::Character::JumpRolled
103 firefall::Character::Respawned
104 firefall::Character::CalledForHelp
105 firefall::Character::TookHit
106 firefall::Character::AlmostHit
107 firefall::Character::DealtHit
108 firefall::Character::Killed
109 firefall::Character::WarnLockTargeted
110 firefall::Character::CurrentPoseUpdate
111 firefall::Character::ConfirmedPoseUpdate
112 firefall::Character::PublicDebugMovementUpdate
113 firefall::Character::ForcedMovement
114 firefall::Character::ForcedMovementCancelled
115 firefall::Character::GrappleClimbPermission
116 firefall::Character::AbilityActivated
117 firefall::Character::AbilityFailed
118 firefall::Character::AbilityCooldowns
119 firefall::Character::NPCInteraction
120 firefall::Character::OpenMovieDialog
121 firefall::Character::PrivateDialog
122 firefall::Character::PublicDialog
123 firefall::Character::AddOrUpdateInteractives
124 firefall::Character::RemoveInteractives
125 firefall::Character::InteractionProgressed
126 firefall::Character::InteractionCompleted
127 firefall::Character::InteractedWithProgressed
128 firefall::Character::InteractedWithCompleted
129 firefall::Character::InventoryUpdate
130 firefall::Character::UnlocksUpdate
131 firefall::Character::WorkbenchUpdate
132 firefall::Character::SimulateLootPickup
133 firefall::Character::DisplayRewards
134 firefall::Character::TrackerEvent
135 firefall::Character::TrackerPulse
136 firefall::Character::PriorityTargetSet
137 firefall::Character::ResourceNodeCompletedEvent
138 firefall::Character::FoundResourceAreas
139 firefall::Character::GeographicalReportResponse
140 firefall::Character::ResourceLocationInfosResponse
141 firefall::Character::UiNamedVariableUpdate
142 firefall::Character::DuelNotification
143 firefall::Character::NewUiQuery
144 firefall::Character::UiQueryCancelled
145 firefall::Character::FetchQueueInfo_Response
146 firefall::Character::MatchQueueResponse
147 firefall::Character::ChallengeCreateResponse
148 firefall::Character::CharacterLoaded
149 firefall::Character::VendorTokenMachineResponse
150 firefall::Character::SalvageResponse
151 firefall::Character::RepairResponse
152 firefall::Character::SlotModuleResponse
153 firefall::Character::UnslotAllModulesResponse
154 firefall::Character::SlotGearResponse
155 firefall::Character::SlotVisualResponse
156 firefall::Character::SlotVisualMultiResponse
157 firefall::Character::TinkeringPlanResponse
158 firefall::Character::UnlockContentSuccess
159 firefall::Character::PushBehavior
160 firefall::Character::PopBehavior
161 firefall::Character::SelfReviveResponse
162 firefall::Character::ApplyCameraShake
163 firefall::Character::ReceivedWebUIMessage
164 firefall::Character::ExitingAttachment
165 firefall::Character::LootDistributionStartEvt
166 firefall::Character::LootDistributionUpdateEvt
167 firefall::Character::LootDistributionCompletionEvt
168 firefall::Character::ForcedWeaponSwap
169 firefall::Character::ChatPartyUpdate
170 firefall::Character::BagInventoryUpdate
171 firefall::Character::LevelUpEvent
172 firefall::Character::FactionReputationUpdate
173 firefall::Character::TutorialStateInitializeEvt
174 firefall::Character::TutorialStateUpdateEvt
175 firefall::Character::DailyLoginRewardsUpdateEvt
176 firefall::Character::Fabrication_FetchAllInstances_Response
177 firefall::Character::Fabrication_FetchAllRecipes_Response
178 firefall::Character::Fabrication_FetchInstance_Response
179 firefall::Character::Fabrication_Start_Response
180 firefall::Character::Fabrication_ApplyAction_Response
181 firefall::Character::Fabrication_GenerateResult_Response
182 firefall::Character::Fabrication_Finalize_Response
183 firefall::Character::Fabrication_Claim_Response
184 firefall::Character::PostStatEvent
185 firefall::Character::BountyRerollProductInfoUpdateEvt
186 firefall::Character::EliteLevels_InitAllFrames
187 firefall::Character::EliteLevels_InitFrame
188 firefall::Character::EliteLevels_UpgradesChanged
189 firefall::Character::EliteLevels_UnusedPointsChanged
190 firefall::Character::EliteLevels_IncreaseXp
191 firefall::Character::EliteLevels_IncreaseLevel
192 firefall::Character::EliteLevels_RerollCompleted
193 firefall::Character::EliteLevels_Initialized_Info
194 firefall::Character::FriendsListChanged
195 firefall::Character::FriendsListResponse
196 firefall::Character::PerkRespecTimerReset
}
case 18 -> 24
Switch(MessageId)
{
83 firefall::AreaVisualData::LootObjectCollected
84 firefall::AreaVisualData::AudioEmitterSpawned
85 firefall::AreaVisualData::ParticleEffectSpawned
}
case 25 -> 30
Switch(MessageId)
{
83 firefall::Vehicle::AbilityActivated
84 firefall::Vehicle::AbilityFailed
85 firefall::Vehicle::PublicCombatLog
86 firefall::Vehicle::CurrentPoseUpdate
87 firefall::Vehicle::TookDebugWeaponHitPublic
88 firefall::Vehicle::ForcedMovement
89 firefall::Vehicle::ForcedMovementCancelled
90 firefall::Vehicle::FlipPunch
91 firefall::Vehicle::DebugMovementUpdate
}
case 33 -> 36
Switch(MessageId)
{
83 firefall::Deployable::TookHit
84 firefall::Deployable::AbilityProjectileFired
85 firefall::Deployable::PublicCombatLog
}
case 37 -> 39
Switch(MessageId)
{
83 firefall::Turret::WeaponProjectileFired
}
case 51 -> 52
Switch(MessageId)
{
83 firefall::LootStoreExtension::LootObjectCollected
}
}
}
else
{
Switch(MessageId)
{
32 firefall::EncounterToUIMessage
33 firefall::VotekickInitiated
34 firefall::VotekickResponded
35 firefall::ScoreBoardEnable
36 firefall::ScoreBoardInit
37 firefall::ScoreBoardSetWinner
38 firefall::ScoreBoardClear
39 firefall::ScoreBoardAddPlayer
40 firefall::ScoreBoardRemovePlayer
41 firefall::ScoreBoardUpdatePlayerStats
42 firefall::ScoreBoardUpdatePlayerStatsFromStats
43 firefall::ScoreBoardUpdatePlayerStatus
44 firefall::MatchLoadingState
45 firefall::MatchEndAck
46 firefall::ServerProfiler_SendNames
47 firefall::ServerProfiler_SendFrame
48 firefall::TempConsoleMessage
49 firefall::ReloadStaticData
50 firefall::EncDebugChatMessage
51 firefall::SendRadioMessage
52 firefall::NpcBehaviorInfo
53 firefall::NpcMonitoringLog
54 firefall::NpcNavigationInfo
55 firefall::NpcHostilityDebugInfo
56 firefall::NpcPositionalDebugInfo
57 firefall::NpcShapeDebugInfo
58 firefall::NpcVoxelInfo
59 firefall::DebugDrawInfo
60 firefall::NpcDevCmdResponse
61 firefall::DevRequestObjectPositions
62 firefall::DevRequestSpawnTables
63 firefall::DevRequestResourceNodeDebug
64 firefall::MissionObjectiveUpdated
65 firefall::MissionStatusChanged
66 firefall::MissionReturnToChanged
67 firefall::MissionsAvailable
68 firefall::MissionActivationAck
69 firefall::BountyStatusChanged
70 firefall::BountyAbortAck
71 firefall::BountyActivationAck
72 firefall::BountyListActiveAck
73 firefall::BountyListActiveDetailsAck
74 firefall::BountyListAvailableAck
75 firefall::BountyClearAck
76 firefall::BountyClearPreviousAck
77 firefall::BountyListPreviousAck
78 firefall::BountyRerollResponse
79 firefall::DisplayUiTrackBounty
80 firefall::Achievements
81 firefall::AchievementUnlocked
82 firefall::TotalAchievementPoints
83 firefall::MissionCompletionCounts
84 firefall::ContentUnlocked
85 firefall::ClientUIAction
86 firefall::ArcCompletionHistoryUpdate
87 firefall::JobLedgerEntriesUpdate
88 firefall::TrackRecipe
89 firefall::ClearTrackedRecipe
90 firefall::SlotTech
91 firefall::InteractableStatusChanged
92 firefall::SendTipMessage
93 firefall::DebugEventSample
94 firefall::DebugLagPlayerSample
95 firefall::DebugLagSimulationSample
96 firefall::DebugLagRaiaSample
97 firefall::DebugEncounterVolumes
98 firefall::Trail
99 firefall::EncounterDebugNotification
100 firefall::EncounterUIScopeIn
101 firefall::EncounterUIUpdate
102 firefall::EncounterUIScopeOut
103 firefall::DisplayUiNotification
104 firefall::DisplayMoneyBombBanner
105 firefall::SetPreloadPosition
106 firefall::PlaySoundId
107 firefall::PlaySoundIdAtLocation
108 firefall::PlayDialogScriptMessage
109 firefall::StopDialogScriptMessage
110 firefall::PingMap
111 firefall::PingMapMarker
112 firefall::EncounterPublicInfo
113 firefall::RequestActiveEncounters_Response
114 firefall::ShoppingListInit
115 firefall::SetClientDailyInfo
116 firefall::GlobalCounterUpdate
117 firefall::GlobalCounterMilestoneInfo
118 firefall::ChatMessageList
119 firefall::CurrentLoadoutResponse
120 firefall::VendorProductsResponse
121 firefall::VendorPurchaseResponse
122 firefall::ConductorGlobalAnnouncement
}
}
83 firefall::Vehicle::Commands::MovementInput
84 firefall::Vehicle::Commands::MovementInputFake
85 firefall::Vehicle::Commands::SinAcquire_Source
86 firefall::Vehicle::Commands::ReceiveCollisionDamage
87 firefall::Vehicle::Commands::ActivateAbility
88 firefall::Vehicle::Commands::DeactivateAbility
89 firefall::Vehicle::Commands::SetWaterLevelAndDesc
90 firefall::Vehicle::Commands::SetEffectsFlag
83 firefall::Turret::Commands::PoseUpdate
84 firefall::Turret::Commands::FireBurst
85 firefall::Turret::Commands::FireEnd
86 firefall::Turret::Commands::FireWeaponProjectile
87 firefall::Turret::Commands::ReportProjectileHit
#####
59 firefall::Encounter::Commands::UiQueryResponse
#####
17 firefall::UIToEncounterMessage
18 firefall::ServerProfiler_RequestNames
19 firefall::ScheduleUpdateRequest
20 firefall::LocalProximityAbilitySuccess
21 firefall::RemoteProximityAbilitySuccess
22 firefall::TrailRequest
23 firefall::RequestLeaveZone
24 firefall::RequestLogout
25 firefall::RequestEncounterInfo
26 firefall::RequestActiveEncounters
27 firefall::VotekickRequest
28 firefall::VotekickResponse
29 firefall::GlobalCounterRequest
30 firefall::CurrentLoadoutRequest
31 firefall::VendorProductRequest
####
83 firefall::Character::Commands::ListItemForSale
84 firefall::Character::Commands::SendMailToPlayer
85 firefall::Character::Commands::FillBuyOrder
86 firefall::Character::Commands::ToggleMarketplace
87 firefall::Character::Commands::JoinGroupLeader
88 firefall::Character::Commands::PlayerReady
89 firefall::Character::Commands::FetchQueueInfo
90 firefall::Character::Commands::MatchQueue
91 firefall::Character::Commands::ClearSavedMatchQueue
92 firefall::Character::Commands::MatchmakerSetPenalties
93 firefall::Character::Commands::MatchAccept
94 firefall::Character::Commands::ChallengeCreate
95 firefall::Character::Commands::ChallengeLeave
96 firefall::Character::Commands::ChallengeInvitation
97 firefall::Character::Commands::ChallengeInvitationResponse
98 firefall::Character::Commands::ChallengeInvitationSquadInfo
99 firefall::Character::Commands::ChallengeKick
100 firefall::Character::Commands::ChallengeSetReady
101 firefall::Character::Commands::ChallengeReadyCheck
102 firefall::Character::Commands::ChallengeSwapTeam
103 firefall::Character::Commands::ChallengeSetRoleAndTeam
104 firefall::Character::Commands::ChallengeSetMatchParameters
105 firefall::Character::Commands::ChallengeSetPowerPrivilege
106 firefall::Character::Commands::ChallengeStartMatch
107 firefall::Character::Commands::LFGCheckin
108 firefall::Character::Commands::LFGLeave
109 firefall::Character::Commands::MapOpened
110 firefall::Character::Commands::BattleframePurchased
111 firefall::Character::Commands::CollectLoot
112 firefall::Character::Commands::TempSlotAbilities
113 firefall::Character::Commands::SinAcquire_Source
114 firefall::Character::Commands::BroadcastWeaponTweaks
115 firefall::Character::Commands::MovementInput
116 firefall::Character::Commands::MovementInputFake
117 firefall::Character::Commands::FireInputIgnored
118 firefall::Character::Commands::FireBurst
119 firefall::Character::Commands::FireEnd
120 firefall::Character::Commands::FireCancel
121 firefall::Character::Commands::FireWeaponProjectile
122 firefall::Character::Commands::ReportProjectileHit
123 firefall::Character::Commands::SelectFireMode
124 firefall::Character::Commands::UseScope
125 firefall::Character::Commands::SelectWeapon
126 firefall::Character::Commands::ReloadWeapon
127 firefall::Character::Commands::CancelReload
128 firefall::Character::Commands::AcquireWeaponTarget
129 firefall::Character::Commands::LoseWeaponTarget
130 firefall::Character::Commands::NPCApplyEffect
131 firefall::Character::Commands::NPCRemoveEffect
132 firefall::Character::Commands::DockToPlayer
133 firefall::Character::Commands::ChangeLookAtTarget
134 firefall::Character::Commands::ActivateAbility
135 firefall::Character::Commands::NPCInteractWithTarget
136 firefall::Character::Commands::TargetAbility
137 firefall::Character::Commands::DeactivateAbility
138 firefall::Character::Commands::ActivateConsumable
139 firefall::Character::Commands::SetNoSpreadFlag
140 firefall::Character::Commands::ExitAttachmentRequest
141 firefall::Character::Commands::NPCSetInteractionType
142 firefall::Character::Commands::PerformEmote
143 firefall::Character::Commands::NotifyDialogScriptComplete
144 firefall::Character::Commands::PerformQuickChatCommand
145 firefall::Character::Commands::PerformTextChat
146 firefall::Character::Commands::PerformDialog
147 firefall::Character::Commands::SetDialogTag
148 firefall::Character::Commands::SetEffectsFlag
149 firefall::Character::Commands::AnimationUpdate
150 firefall::Character::Commands::SelectLoadout
151 firefall::Character::Commands::CallForHelp
152 firefall::Character::Commands::AbortCampaignMission
153 firefall::Character::Commands::TryResumeTutorialChain
154 firefall::Character::Commands::DebugMission
155 firefall::Character::Commands::AssignBounties
156 firefall::Character::Commands::AbortBounty
157 firefall::Character::Commands::ActivateBounty
158 firefall::Character::Commands::ListActiveBounties
159 firefall::Character::Commands::ListActiveBountyDetails
160 firefall::Character::Commands::ListAvailableBounties
161 firefall::Character::Commands::ClearBounties
162 firefall::Character::Commands::ClearPreviousBounties
163 firefall::Character::Commands::ListPreviousBounties
164 firefall::Character::Commands::RequestRerollBounties
165 firefall::Character::Commands::TrackBounty
166 firefall::Character::Commands::SetBountyVar
167 firefall::Character::Commands::RefreshBounties
168 firefall::Character::Commands::ListAchievements
169 firefall::Character::Commands::RequestAchievementStatus
170 firefall::Character::Commands::RequestAllAchievements
171 firefall::Character::Commands::RequestMissionAvailability
172 firefall::Character::Commands::RequestNewActivity
173 firefall::Character::Commands::RequestPushMission
174 firefall::Character::Commands::LogDirectActivityRequest
175 firefall::Character::Commands::LogActivityPush
176 firefall::Character::Commands::LogLongTimeWithoutPush
177 firefall::Character::Commands::CameraPoseUpdate
178 firefall::Character::Commands::QueueUnstuck
179 firefall::Character::Commands::VehicleCalldownRequest
180 firefall::Character::Commands::DeployableCalldownRequest
181 firefall::Character::Commands::DeployableHardpointSelection
182 firefall::Character::Commands::ResourceNodeBeaconCalldownRequest
183 firefall::Character::Commands::FindNearbyResourceAreas
184 firefall::Character::Commands::GeographicalReportRequest
185 firefall::Character::Commands::UpdateChatPartyMembers
186 firefall::Character::Commands::ClientQueryInteractionStatus
187 firefall::Character::Commands::ResourceLocationInfosRequest
188 firefall::Character::Commands::DuelRequest
189 firefall::Character::Commands::PickupCarryableObjectByProximity
190 firefall::Character::Commands::DropCarryableObject
191 firefall::Character::Commands::AiError
192 firefall::Character::Commands::AiSignal
193 firefall::Character::Commands::NonDevDebugCommand
194 firefall::Character::Commands::UpdateShoppingList
195 firefall::Character::Commands::FindServiceProvider
196 firefall::Character::Commands::ClientUIEvent
197 firefall::Character::Commands::SetMovementSimulation
198 firefall::Character::Commands::RequestRespawn
199 firefall::Character::Commands::RequestTransfer
200 firefall::Character::Commands::VendorTokenMachineRequest
201 firefall::Character::Commands::TimedDailyRewardRequest
202 firefall::Character::Commands::SalvageRequest
203 firefall::Character::Commands::RepairRequest
204 firefall::Character::Commands::SlotModuleRequest
205 firefall::Character::Commands::UnslotAllModulesRequest
206 firefall::Character::Commands::SlotGearRequest
207 firefall::Character::Commands::SlotVisualRequest
208 firefall::Character::Commands::SlotVisualMultiRequest
209 firefall::Character::Commands::RequestSelfRevive
210 firefall::Character::Commands::RequestTeleport
211 firefall::Character::Commands::RequestFrameLevelReset
212 firefall::Character::Commands::LeaveEncounterParty
213 firefall::Character::Commands::JoinSquadLeadersArc
214 firefall::Character::Commands::LeaveArc
215 firefall::Character::Commands::JobLedgerOperation
216 firefall::Character::Commands::SeatChangeRequest
217 firefall::Character::Commands::RequestTrackerUpdate
218 firefall::Character::Commands::LootDistributionSetState
219 firefall::Character::Commands::LootDistributionSetVotes
220 firefall::Character::Commands::ResetTutorialId
221 firefall::Character::Commands::DismissTutorialId
222 firefall::Character::Commands::ClaimDailyRewardItem
223 firefall::Character::Commands::ClaimDailyRewardStreak
224 firefall::Character::Commands::BuyBackPreviousDay
225 firefall::Character::Commands::AcceptRewards
226 firefall::Character::Commands::FlushRewards
227 firefall::Character::Commands::RerollEliteLevelsAwardList
228 firefall::Character::Commands::RevertAllEliteLevelsUpgrades
229 firefall::Character::Commands::SelectEliteLevelsAward
230 firefall::Character::Commands::ResetAllEliteLevelsUpgrades_Debug
231 firefall::Character::Commands::FlushCharacterCache
232 firefall::Character::Commands::RunTeamManagerCommand
233 firefall::Character::Commands::NPCCombatUpdate
234 firefall::Character::Commands::BagInventorySettings
235 firefall::Character::Commands::SetSteamUserId
236 firefall::Character::Commands::EquipExperimentalLoadout
237 firefall::Character::Commands::ExecuteTinkeringPlan
238 firefall::Character::Commands::VendorPurchaseRequest
239 firefall::Character::Commands::TutorialEventTriggeredCmd
240 firefall::Character::Commands::Fabrication_FetchAllInstances
241 firefall::Character::Commands::Fabrication_FetchAllRecipes
242 firefall::Character::Commands::Fabrication_FetchInstance
243 firefall::Character::Commands::Fabrication_Start
244 firefall::Character::Commands::Fabrication_ApplyAction
245 firefall::Character::Commands::Fabrication_GenerateResult
246 firefall::Character::Commands::Fabrication_Finalize
247 firefall::Character::Commands::Fabrication_Claim
248 firefall::Character::Commands::FriendsListRequest
249 firefall::Character::Commands::UpdateFriendStatus
250 firefall::Character::Commands::ClaimBountyRewards
59 firefall::Character::Commands::UiQueryResponse
Channel 0
Channel 0 seems to be for protocol level control commands such as ACK's, NACK's and time updates.
After the previous packet header channel 0 only adds a byte as the message id, after this is per packet data.
I won't list some of the channel 0 packet formats here to save some space.
Channel 1
Channel 1 just adds a byte
for the message id after the previous header and everything else after that is per packet data.
These packets seem to be for the more abstarct game functions that don't directly relate to in world entitys.
Below is the packet names for each known packet:
click to show
Channel 1 Packet ids to names
17 matrix_fury::Login
18 matrix_fury::EnterZoneAck
19 matrix_fury::ExitZoneAck
20 matrix_fury::KeyframeRequest
21 matrix_fury::DEV_ExecuteCommand
22 matrix_fury::Referee_ExecuteCommand
23 matrix_fury::RequestPause
24 matrix_fury::RequestResume
25 matrix_fury::ClientStatus
26 matrix_fury::ClientPreferences
27 matrix_fury::SynchronizationResponse
28 matrix_fury::SuperPing
29 matrix_fury::StressTestMasterObject
30 matrix_fury::ServerProfiler_RequestNames
31 matrix_fury::LogInstrumentation
32 matrix_fury::RequestSigscan
33 matrix_fury::SendEmergencyChat
34 matrix_fury::SigscanData
35 matrix_fury::WelcomeToTheMatrix
36 matrix_fury::Announce
37 matrix_fury::cakoneone
38 matrix_fury::UpdateZoneTimeSync
39 matrix_fury::HotfixLevelChanged
40 matrix_fury::ExitZone
41 matrix_fury::MatrixStatus
42 matrix_fury::MatchQueueResponse
43 matrix_fury::MatchQueueUpdate
44 matrix_fury::FoundMatchUpdate
45 matrix_fury::ChallengeJoinResponse
46 matrix_fury::ChallengeInvitation
47 matrix_fury::ChallengeInvitationSquadInfoAck
48 matrix_fury::ChallengeInvitationCancel
49 matrix_fury::ChallengeInvitationResponse
50 matrix_fury::ChallengeKicked
51 matrix_fury::ChallengeLeave
52 matrix_fury::ChallengeRosterUpdate
53 matrix_fury::ChallengeReadyCheck
54 matrix_fury::ChallengeMatchParametersUpdate
55 matrix_fury::ChallengeMatchStarting
56 matrix_fury::ForceUnqueue
57 matrix_fury::SynchronizationRequest
58 matrix_fury::GamePaused
59 matrix_fury::SuperPong
60 matrix_fury::ServerProfiler_SendNames
61 matrix_fury::ServerProfiler_SendFrame
62 matrix_fury::ZoneQueueUpdate
63 matrix_fury::DebugLagSampleSim
64 matrix_fury::DebugLagSampleClient
65 matrix_fury::LFGMatchFound
66 matrix_fury::LFGLeaderChange
67 matrix_fury::ReceiveEmergencyChat
68 matrix_fury::UpdateDevZoneInfo
Channel 3
If a message sent in this channel get too long and needs to be split it will instead be split up and sent in channel 2 as a reliable packet. (The client emits warning in cases like this)
Channel 3 is much like channel 2 but unreliable.
Packet parsing overview diagram
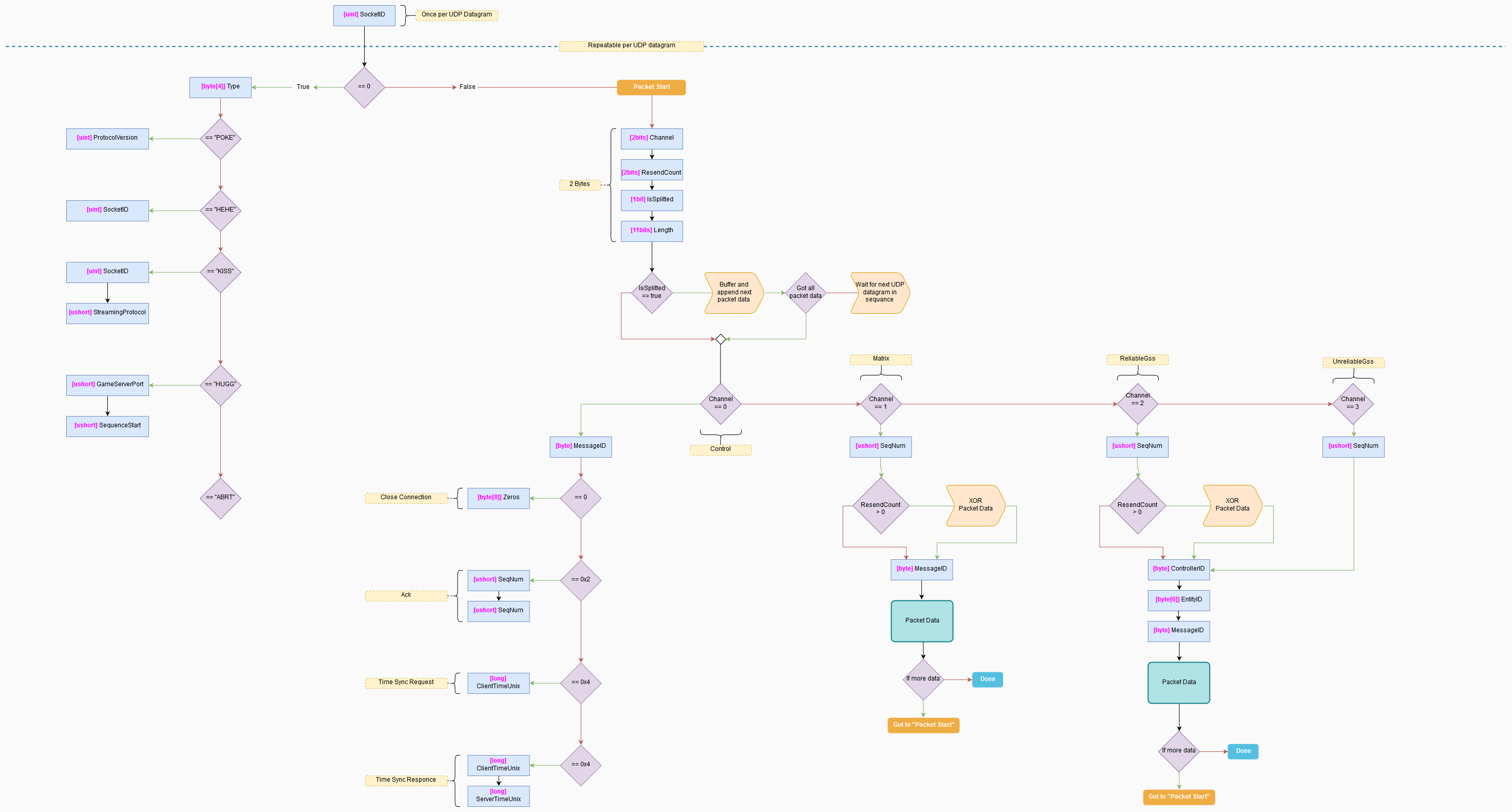
Todos and whats next:
- Verify some of the assumptions that are made above.
- Iron out some of the smaller details, eg handling droped packets.
- Finish libary for handling the low level protocol stuff so we can focus on the packet contents and have a good framework for writing parsers for them.
- Documentation for for those packet formats.
- More tooling for exploring packet captures nicely (PacketPeep and 010 Buddy).
- Formating and feed back on restructing this so that it is easyier to read and understand.